这篇论文发表于2021.10,大部分内容在官网上,对一些重点内容做一下记录。
I. INTRODUCTION
算法框架包括探索算法TARE
(exploration planner
); 路径算法FAR
(route planner
)
我们的算法不依赖只有仿真才提供的信息,比如 semantic and terrain segmentation groundtruth. 算法是为了让用户重点开发上层的规划算法。
在2021 DARPA地下挑战赛中, CMU-OSU Team Explorer 虽然没拿到冠军,但获得”探索最多区域奖“(26 out of 28 sectors).
For outdoor settings, the most well-known dataset is KITTI Vision Benchmark Dataset
[4] collected
from a self-driving suite in road driving scenarios. The
dataset contains sensor data from stereo cameras, 3D Lidar,
and GPS/INS ground truth for benchmarking depth reconstruction, odometry estimation, object segmentation, etc.
For localization purposes, the long-term localization benchmark includes a comprehensive list of datasets: Aachen Day-Night dataset, Extended CMU Seasons dataset, RobotCar Seasons dataset, and SILDa Weather and Time of Day dataset.
For indoor navigation, iGibson [15], [16], Sapien [17], AI2Thor [18], Virtual Home [19], ThreeDWorld [20], MINOS [21], House3D [22] and CHALET [23] use synthetic scenes, while reconstructed scenes are also available in iGibson, AI Habitatand MINOS. Compared to datasets, simulation environments have the advantage of providing access to ground truth data, e.g. vehicle pose and semantic segmentation to simplify the algorithm development and allowing full navigation system tests in closed control loop。
For indoor scenes, datasets such as NYUDepth dataset [7], TUM SLAM dataset [8], inLoc dataset [9], MIT Stata center dataset, and KTH-INDOL dataset [11], [12] are available. Datasets are useful in developing and
Simulation environments: Carla [13] and AirSim [14] are two representative simulation environments for autonomous driving and flying. These simulators support various conditions such as lighting and weather changes, moving objects such as pedestrians, and incident scenes.
其他的导航框架: ROS Navigation Stack 和港科大的 fast_planner ,不过是针对无人机的。
我们的导航框架: 支持photorealistic house models from Matterport3D 以及向AI Habitat提供接口。这两个在机器人学和计算视觉中应用很多。Users are provided with scan data and RGB, depth, and semantic images rendered by AI Habitat. Users have the option of running AI Habitat side by side with our system or in post-processing

参考:
阅读笔记 《Matterport3D: Learning from RGB-D Data inIndoor Environments》
AI Habitat室内仿真平台使用
III. DEVELOPMENT ENVIRONMENT
Local Planner
local planner
保证了到达目标时的安全,它预计算 a motion primitive library and associates the motion primitives to 车周围的3D locations(这里说的就是free_paths
对应的曲线簇)。 The motion primitives are modeled as Monte Carlo samples and organized in groups
现实中快遇到障碍物时,local planner
可以在几毫秒内判断出哪些 motion primitives 会和障碍相撞,然后从motion primitives中选出最可能朝向目标的一组。
The module also has interface to take in additional range data for collision avoidance as an extension option
Terrain Traversability Analysis
模块建立了一个代价地图,地图中的每一个点和一个traversal cost相关联. 代价值取决于 local smoothness of the terrain.
我们使用体素网格表示环境,分析 distributions of data points in adjacent voxels to 估计地面高度. The points are associated with higher traversal costs if they are further apart from the ground.
模块还能处理negative obstacles
,这些障碍生成空区域with no data points on the terrain map. 如果开启了negative obstacle
的处理,模块认为那些区域不可经过
可视化算法的性能. 可视化工具显示地图,已探索区域,车的轨迹. Metrics包括 explored volume, traveling distance, and algorithm runtime are plotted and logged to files.
另外,支持手柄和导航交互,在多种操作模式之间切换 to ease the process of system debugging.
IV. HIGH-LEVEL PLANNERS
V. BEST PRACTICES
Safety margin: local planner
使用车和waypoint
之间的距离作为规划尺度(planning horizon)。即使waypoint
很接近障碍,也可以让车到达。但是,如果车不能在waypoint
停下,上层规划器倾向于让车到waypoint
保持一定距离(默认≥
3.75m)。If the waypoint is closer, users can project the waypoint further away and keep the waypoint in the same direction w.r.t. the vehicle to fully use the safety margin. (这句话没理解什么意思)
但是,如果车需要经过窄通道,降低这一距离可以让local planner
找到安全合适的路径。
大转弯: Typically, a high-level planner selects the waypoint along the path that is a distance, namely look-ahead distance, ahead of the vehicle and sends the waypoint to the local planner (possibly after projecting the waypoint further away from the vehicle as discussed above).
When handling sharp turns (≥ 90 deg), the look-ahead distance needs to be properly set or the waypoint may jump to the back of the vehicle, causing the vehicle to osculate back-and-forth. We recommend to select the waypoint on the starting segment of the path that is in line-of-sight from the vehicle.
动态障碍: terrain analysis
模块在动态障碍走过之后,通过ray-tracing
清除动态障碍。这是在车的附近范围实现的(到车的距离≤5m), 因为雷达数据在远处比较稀疏,以及难以权衡清除动态障碍和thin structures之间的矛盾。 用户根据自己的情况来清除动态障碍,TARE和FAR都有这一步骤。
VI. SYSTEM INTEGRATION
用于DARPA挑战赛的平台
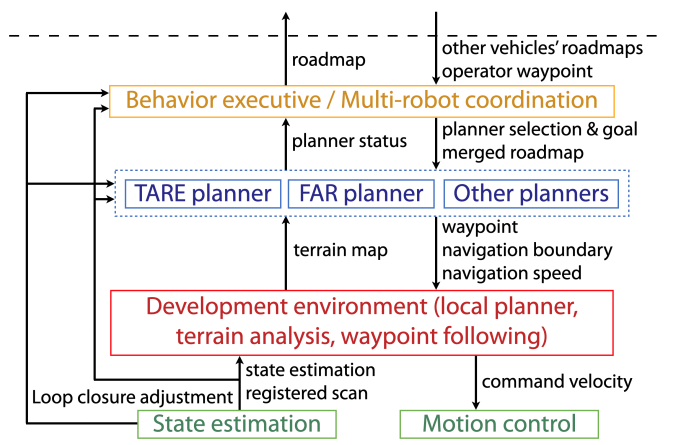
The state estimation module can detect and introduce loop closures. The module outputs state estimation in the odometry frame generated by 3D Lidar-based odometry containing accumulated drift. When loop closure is triggered, it outputs loop closure adjustments to globally relax the vehicle trajectory and corresponding maps. Loop closure adjustments are used by the high-level planners since they are in charge of planning at the global scale.
The local planner and terrain analysis modules are extended to handle complex terrains including negative obstacles such as cliffs, pits, and water puddles with a downward-looking depth sensor. The TARE planner, FAR planner, and other planners (for stuck recovery, etc) are run in parallel for tasks such as exploration, go-to waypoint, and return home.
On top of these planners, behavior executive and multi-robot coordination modules are built specifically for the challenge. The modules share explored and unexplored areas across multirobots and call TARE and FAR planners cooperatively. In particular, when a long-distance transition is determined due to new areas containing artifacts are discovered or operator waypoints are received, the behavior executive switches to
FAR planner for relocating the vehicle. During the relocation, FAR planner uses a sparse roadmap merged from multi-robots for high-level guidance. After the relocation is complete, TARE planner takes place for exploration
DARPA最后决赛是在Louisville Mega Cavern, KY
. 赛道包括隧道、urban、洞穴环境。我们的三台车使用TARE
和 FAR
进行探索。
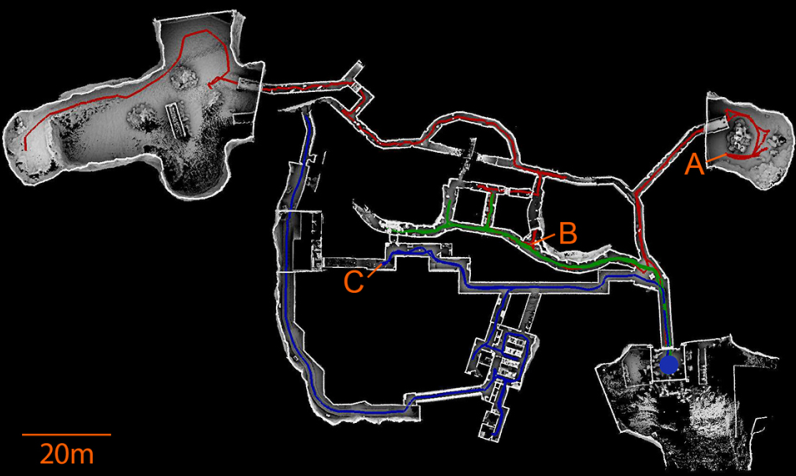
红色轨迹:车先使用TARE一直探索到B,然后使用FAR经过C,一到达C,车换成TARE进行剩下的探索。
绿色轨迹:车只用TARE;蓝色轨迹:车先使用FAR经过A,在换成TARE进行之后的搜索。
三台车各行走596.6m, 499.8m, 445.2m。共花费时间2259s
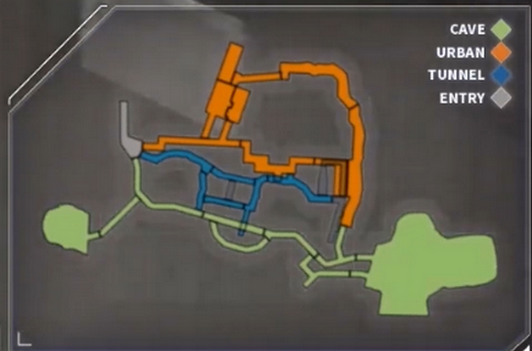
这里只看论文不太明白,看实际视频才清楚。实际是两辆车和一架无人机进行探索。
VII. EXTENDED APPLICATION EXAMPLES
这部分我暂时用不到,就不研究了。
训练基于学习的探索和导航算法。大家可以使用我们的仿真平台作为专家系统(expert system)来训练基于学习的规划算法。专家系统里包含状态估计,局部避障,上层的探索或者路径规划算法。训练的对象可以是一些端对端的方法,比如只有一层深度网络的探索或者路径规划算法。
基于激光或者视觉的sim-to-real学习。大家可以用我们的仿真平台训练一些基于学习的方法,并将训练好的系统应用在现实世界中。因为我们提供了多个仿真世界以及支持来自Matterport3D的90个photorealistic环境模型,并且兼容AI Habitat的RGB,深度,和语义(Semantic)图像的渲染功能,所以不管是基于激光还是视觉的算法,大家都可以找到适用的环境模型,当然也可以根据需求换上自己的模型。举个简单的例子,需要做Semantic-in-loop导航的人可以使用我们提供的带有语义信息的环境,使用我们的地面机器人仿真平台,去训练他们的算法,并且在训练结束后,直接将算法移植到实际的机器人上。下面是我们的仿真机器人在Matterport3D的两个环境模型中导航的场景,用AI Habitat实时生成RGB,深度及语义图像。
开发人群导航(social navigation)算法。大家可以在我们的仿真环境中加入动态的障碍物或者行人,模拟真实环境中的社区场景,辅助开发人群导航算法,甚至可以在这种场景中预测动态物体的行为及其轨迹。